Code-It-a-idangal
Monday, March 5, 2012
Technical Debt
Either the culprit :P or victim :(.
It is basically the technical things that we say "WE WILL DO IT LATER".. Or in a simple terms the pending "TODOs" ..
> It could be a misspell of variable name in various source files.
> It could be unorganized imports in a java source.
> No unit test cases => most common one.
> No proper documentation, sometimes not even comments..
The reasons could be anything starting from carelessness to project pressure :)..
Some of them are unintentionally , some intentional, former is fine we cannot track[only reviews can help], latter is something interesting to be discussed.
I came across this interesting article
http://blogs.construx.com/blogs/stevemcc/archive/2007/11/01/technical-debt-2.aspx
Enjoy reading
Thursday, April 28, 2011
Using Selenium for System and Regression Testing
Dears,
For past few days I was exploring the tool selenium it is very popular in the agile world for testing web applications. To describe simply:
- Selenium can record our web activities [Currently supported only as firefox addon].
- Recorded testcases can be saved in different languages [in our case Java as JUnit testcase].
- Testsuites of these testcases can be run on any browsers automatically.[In our case Internet Explorer].
Recording:
Selenium IDE installation and use:
- Install the Firefox addon, [selenium-ide-1.0.4.xpi]
- Open URL www.google.com in firefox.
- Click on tools-> Selenium IDE
Change Base URL textbox to reflect http://www.google.com/ if its not the case already.
- Move your mouse the RED round icon at the top-right corner. [Click on it if it is not already recording].
- Switch to google search window. Search for text Infosys
- Right click any link in that results page and select verifyText present context menu item
- Switch back to the selenium IDE window. You will notice the steps are recorded by selenium.
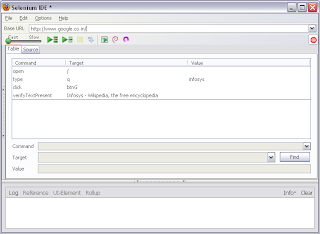
- Click on the red button to stop recording. If you want you can just run the test case by pressing the green Arrow buttons in the toolbar of the window.
- Now click File->Export testcase As in Selenium IDE and select Java[JUnit] – Selenium RC
- Save this with .java extension. You must has a file like
package com.example.tests;
import com.thoughtworks.selenium.*;
import java.util.regex.Pattern;
public class Untitled extends SeleneseTestCase {
public void setUp() throws Exception {
setUp("http://www.google.co.in/", "*chrome");
}
public void testUntitled() throws Exception {
selenium.open("/");
selenium.type("q", "infosys");
selenium.click("btnG");
verifyTrue(selenium.isTextPresent("Infosys - Wikipedia, the free encyclopedia"));
}
}
This is the testcase which can be run automatically, we can customize the parameters and flow. Below I will explain to automatically run this recorded testcase in Internet explorer.
Running Remote Control:
- For this we need to have selenium RC [remote control].
- Get the same from selenium-remote-control-1.0.1-dist.zip
- Unzip this in any location in your PC.
- Open command prompt and change directory to location <Unzipped Location>\selenium-remote-control-1.0.1-dist\selenium-remote-control-1.0.1\selenium-server-1.0.1
- Now run java -jar selenium-server.jar [note you are in the location where the .jar file is present]
- You will see something like
C:\Narain\0_Applications\selenium-remote-control-1.0.1-dist\selenium-remote-control-1.0.1\selenium-server-1.0.1>java -jar selenium-server.jar
14:32:02.218 INFO - Java: Sun Microsystems Inc. 1.5.0_15-b04
14:32:02.218 INFO - OS: Windows XP 5.1 x86
14:32:02.264 INFO - v1.0.1 [2696], with Core v@VERSION@ [@REVISION@]
14:32:02.374 INFO - Version Jetty/5.1.x
14:32:02.374 INFO - Started HttpContext[/,/]
14:32:02.374 INFO - Started HttpContext[/selenium-server,/selenium-server]
14:32:02.374 INFO - Started HttpContext[/selenium-server/driver,/selenium-server/driver]
14:32:02.421 INFO - Started SocketListener on 0.0.0.0:4444
14:32:02.421 INFO - Started org.mortbay.jetty.Server@6b7920
- This means selenium RC is running.
Running Test Cases:
- Create new java project in eclipse.
- Add the junit and Selenium lib files junit-4.8.1.jar and selenium-java-client-driver.jar to the buildPath
- Just copy the saved java file into this project.
- Right click and run as Junit test case.
I assume above gave you a introduction to the tool. You can google for more information. I have used this to run a complexusecase for all the customer in database in less than a minute and also I have customized to take screenshots for each page load eg.,
selenium.select("dbo_searchBy", "label=" + SearchBy);
selenium.type("searchByValue", searchByValue);
selenium.captureScreenshot("C:/Narain/1_Projects/1_GLP/5_Development/Sprint2/FunctionalTesting/Captures2/" + searchByValue +"SearchScreen.png");
selenium.click("checkEliBtn");
selenium.waitForPageToLoad("30000");
selenium.click("checkEliBtn");
selenium.waitForPopUp("CustomerComposition", "30000");
selenium.captureScreenshot("C:/Narain/1_Projects/1_GLP/5_Development/Sprint2/FunctionalTesting/Captures2/" + searchByValue +"CustomerComposition.png");
This way the testcases once recorded and customized can be run for several customers and can be run as regression when new functionalities are developed. I am working on automating all this using ANT and HUDSON to automatically build, deploy and test.
Thanks for your patience for reading this long Blog!
Tuesday, March 15, 2011
Java Unit testing
Java Unit Testing:
1. Tool: JUnit.
2. Process:
a. Sources has the new folder structure added for this NGRP->UnitTesting.
b. Use Eclipse to generate the unit test skeleton for your target class [All the screenshots are self explanative] ->>>> Look at the folder structure in clearcase
Naming Convention: <TargetClass>Test
Choose target method to test choose checkboxes as below
Note: Never add the cases to clearcase before testing locally. If eclipse plugin claims just cancel the dialog.
c. Most of the cases it is not as straight forward to write unit test , sometimes methods calls other methods in different layer.
To unit test such methods we should start doing Refactoring.
Target Class:
class TestActionClass {
...
public subscriptionTO callRules(subscriptionTO) {
if (level=”G”){
subscriptionTO.level=”G”;
}
elseif(level=”A”){
subscriptionTO.level=”A”;
}
RemoteService r = new RemoteService();
r.callRules(subscriptionTO);
return subscriptionTO;
}
..
}
With the above example you cannot unit test as the function call will try to call RemoteService which needs server to be running and ofcourse application to be deployed.
Action-> Refactor the call to Remote method to make this method[callRemote] unit testable:
Refactored Class:
class TestActionClass {
...
public subscriptionTO callRules(subscriptionTO) {
if (level=”G”){
subscriptionTO.level=”G”;
}
elseif(level=”A”){
subscriptionTO.level=”A”;
}
RemoteService r = createRemoteService();
r.callRules(subscriptionTO);
return subscriptionTO;
}
..
protected RemoteService createRemoteService(){
return new RemoteService();
}
}
Now you can write a unit Testcase as follows:
Unit Test Class:
class TestActionClassTest {
MYObject sampleObject = new MYObject();
...
@Before
public void setUp() throws Exception {
sampleObject.level=”G”;
}
@Test
public void testCallRules() {
TestActionClass t = TestActionClass(){
protected RemoteService createRemoteService(){
return sampleObject;
}
};
}
..
}
As you can see above now I have overridden the createRemoteService method in my unit testcase, thus passing my mock object to test callRemote and avoid calling other layers.
Reference: http://www.ibm.com/developerworks/library/j-mocktest.html
Saturday, May 29, 2010
Zig - Chapter I
Shocking?.. Yes Development is not the first phase but I intentionally skipped first few phases and jumped straight to development.
On hearing the word development, First few points that strikes our head may be listed as
1. Coding Technology[Language]
2. Coding Standards
3. Source Control
4. IDE to use
Readers just note my post will just talk about Java and Oracle..May be eventually about IBM Websphere JRules.
Java: The programming language I hated the most during my college days, but now it has become heart throb. Yes I started loving it for the very good reason that it is "Lovable" :)
Now even school students learn java. I even heard few do freelancing !!!.
Experience YES X->Perience
People name it "Learning Curve", I would say its very very zig-zagged in my case. Yes I just went through various little interesting learnings in professional life. I always like sharing my sweet incidents in my personal life with people around me, in relevant or even irrelevant situations. But rarely do share my professional life. So if you are still reading, you would have guessed by this time, that I am going to blog the same and ofcourse it will be fun for me to read later. With my 5+ years of experience in infosys, I am going to define or paraphrase down various stages of SDLC, in my style and way I worked.
First interesting stuff is the task board